Setting up Flask SQLAlchemy with PostgreSQL
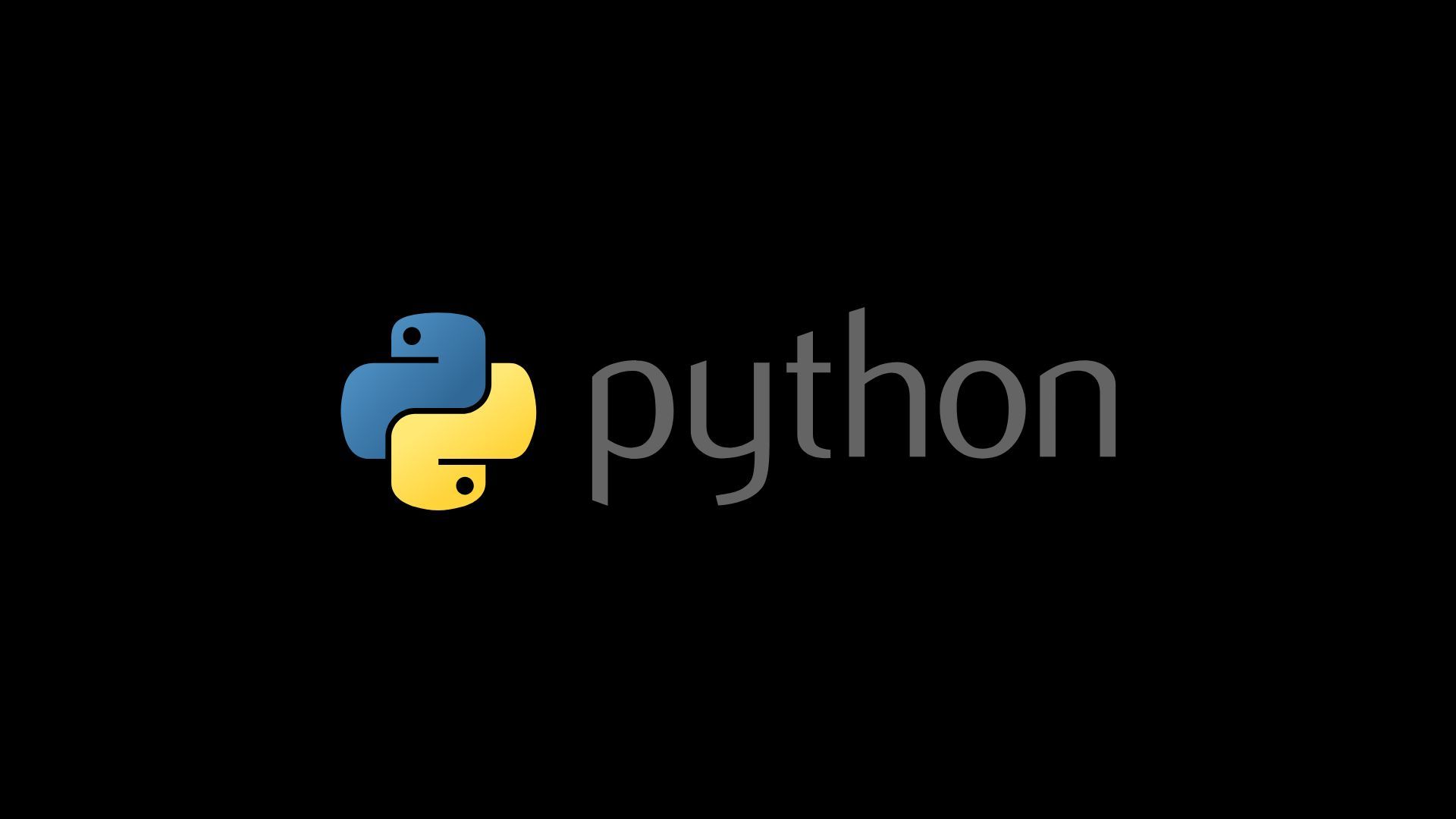
Add these packages to your requirements.txt
:
flask
Flask-SQLAlchemy
psycopg2-binary
Then install them from your terminal
pip install -r requirements.txt
In main.py
:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
from flask_cors import CORS
# database connection parameters
DB_HOST = 'localhost'
DB_PORT = 5432
DB_USER = 'me'
DB_PASSWORD = 'password'
DB_DATABASE = 'mydb'
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = f"postgresql://{DB_USER}:{DB_PASSWORD}@{DB_HOST}:{DB_PORT}/{DB_DATABASE}"
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Person(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(), nullable=False)
# this function lets you convert an instance of this class into a dictionary
def as_dict(self):
return {c.name: getattr(self, c.name) for c in self.__table__.columns}
@app.route('/', methods=['GET'])
def index():
return 'Hello world!'
if __name__ == '__main__':
with app.app_context():
db.create_all() # create tables
app.run(port=8000, host='0.0.0.0')
Finally, run app with python main.py