Serving a static folder in Python Flask
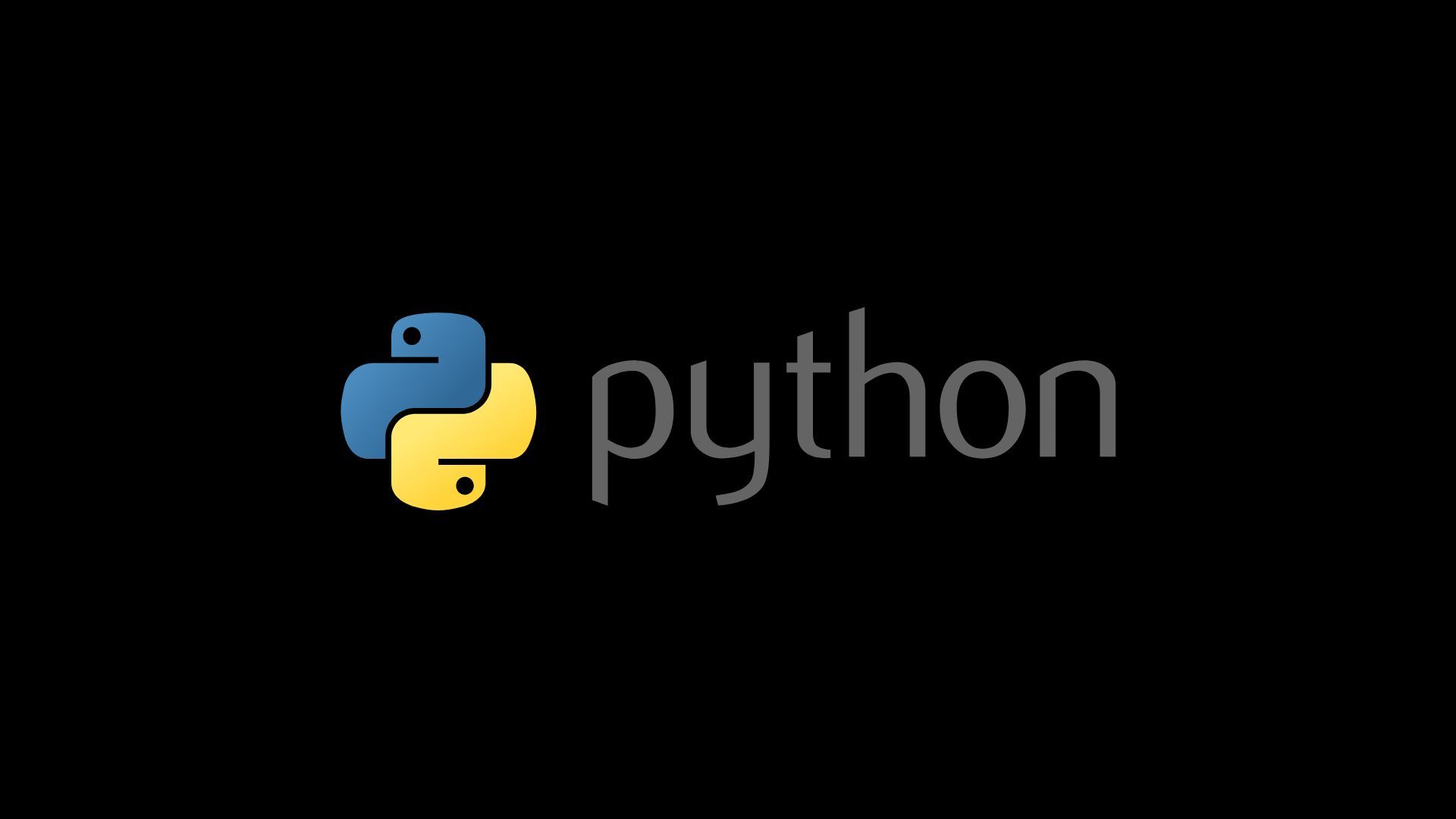
Serving a folder named static
:
from flask import Flask, send_from_directory
app = Flask(__name__)
@app.route('/static/<path:path>')
def static_assets(path):
return send_from_directory('static', path)
Serving a built frontend project (eg. Vue, React)
In this example, the built project in folder dist
will be served, and all paths not found in the folder will be directed to index.html
to accomodate page-history router functionality in SPAs.
from flask import Flask, send_from_directory
from os.path import exists
@app.route('/', defaults={'path': ''})
@app.route('/<path:path>')
def catch_all(path):
if not path or not exists(f'../dist/{path}'):
return send_from_directory('../dist', 'index.html')
folder = '../dist'
path_chunks = path.split('/')
if len(path_chunks[-1]) > 1:
folder += '/' + '/'.join(path_chunks[:-1])
return send_from_directory(folder, path_chunks[-1])