How to setup Redux in a React project
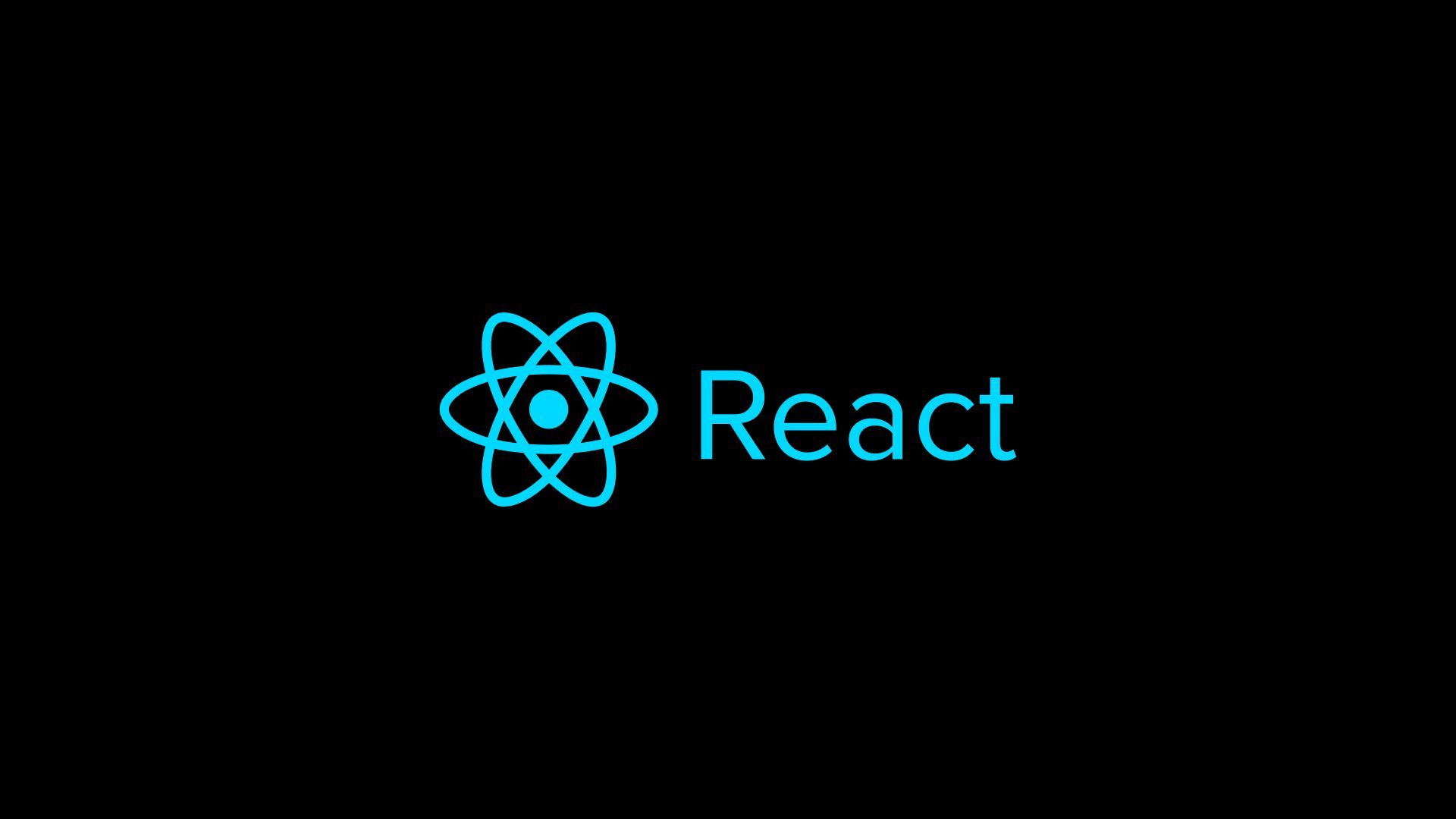
Installing Redux:
npm install @reduxjs/toolkit react-redux
Create a src/store
folder. We will create a numeric counter in our state, Redux calls this a “slice” of the state. To create our slice, create src/store/counter.jsx
. Each slice should live in a file similar to this one.
// src/store/counter.jsx
import { createSlice } from '@reduxjs/toolkit'
const counter = createSlice({
name: 'counter',
initialState: {
value: 0,
},
reducers: {
increment: (state) => {
state.value += 1
},
decrement: (state) => {
state.value -= 1
},
incrementByAmount: (state, action) => {
state.value += action.payload
},
},
})
export const { increment, decrement, incrementByAmount } = counter.actions
export default counter.reducer
Create src/store/index.jsx
file, this will be our main store. Import the counter we created earlier, and add it to our store.
// src/store/index.jsx
import { configureStore } from '@reduxjs/toolkit'
import counter from "./counter.jsx";
export default configureStore({
reducer: {
counter,
}
})
Finally, in src/main.jsx
, import the store and wrap Redux’s context provider around our app:
// src/main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import './index.css'
import App from './App'
import store from './store' // add this line
import { Provider } from 'react-redux' // add this line
const root = ReactDOM.createRoot(document.getElementById('root')).render(
<Provider store={store}> {/* add this line */}
<App />
</Provider> {/* add this line */}
)
Using the store
To use and modify the count in your components:
Installing Redux:
npm install @reduxjs/toolkit react-redux
Create a src/store
folder. We will create a numeric counter in our state, Redux calls this a “slice” of the state. To create our slice, create src/store/counter.jsx
. Each slice should live in a file similar to this one.
// src/store/counter.jsx
import { createSlice } from '@reduxjs/toolkit'
const counter = createSlice({
name: 'counter',
initialState: {
value: 0,
},
reducers: {
increment: (state) => {
state.value += 1
},
decrement: (state) => {
state.value -= 1
},
incrementByAmount: (state, action) => {
state.value += action.payload
},
},
})
export const { increment, decrement, incrementByAmount } = counter.actions
export default counter.reducer
Create src/store/index.jsx
file, this will be our main store. Import the counter we created earlier, and add it to our store.
// src/store/index.jsx
import { configureStore } from '@reduxjs/toolkit'
import counter from "./counter.jsx";
export default configureStore({
reducer: {
counter,
}
})
Finally, in src/main.jsx
, import the store and wrap Redux’s context provider around our app:
// src/main.jsx
import React from 'react'
import ReactDOM from 'react-dom/client'
import './index.css'
import App from './App'
import store from './store' // add this line
import { Provider } from 'react-redux' // add this line
const root = ReactDOM.createRoot(document.getElementById('root')).render(
<Provider store={store}> {/* add this line */}
<App />
</Provider> {/* add this line */}
)
Using the store
To use and modify the count in your components: