How to set up image uploads in Python Flask
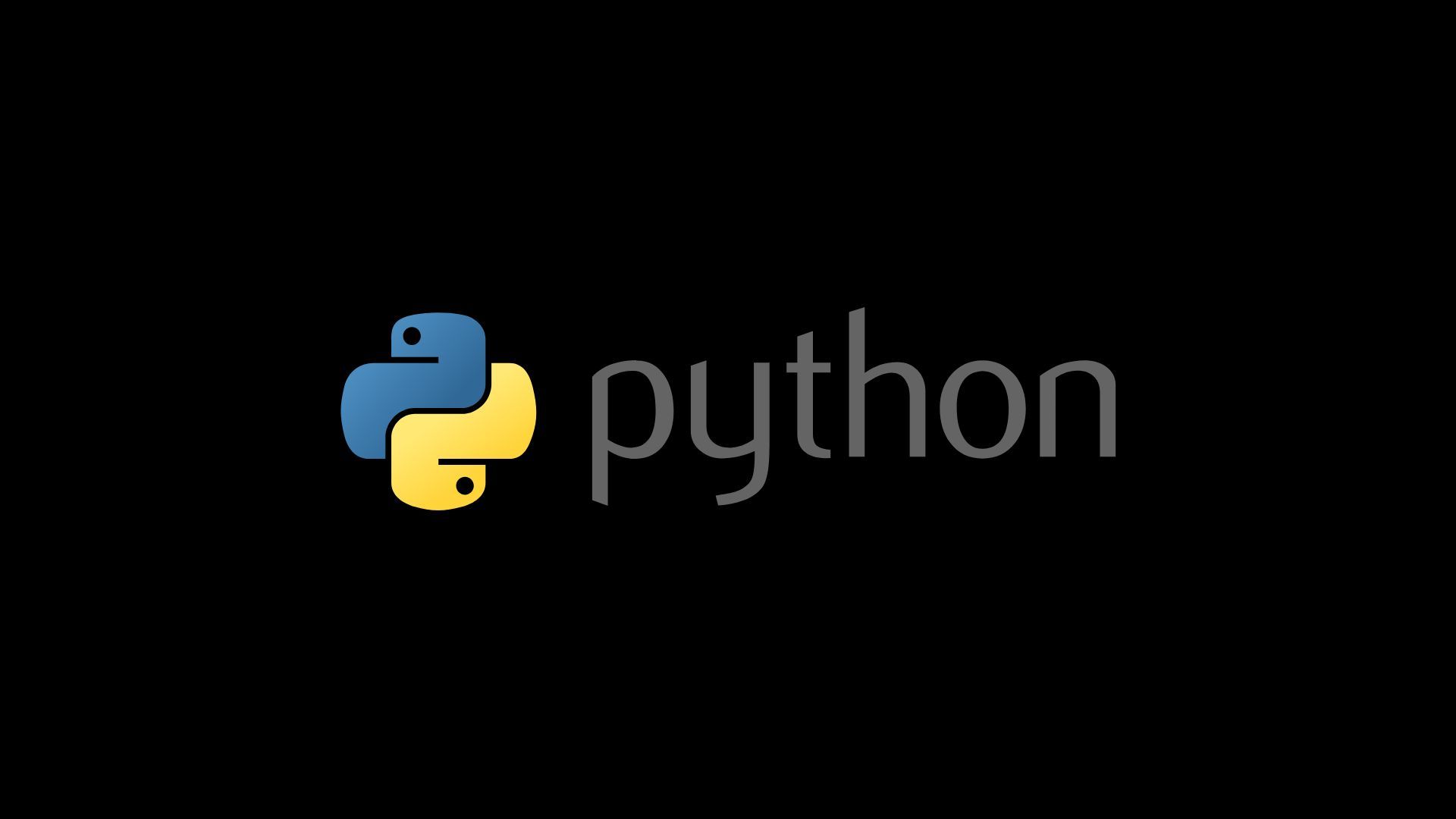
Set up a POST route to accept an image
field:
import uuid
import os
from flask import request, Response
# specify the path where you want to keep the uploaded files
IMAGE_UPLOADS = './filestore'
@app.route('/upload_img', methods=['POST'])
def upload_img():
if request.method == 'POST' and request.files:
image = request.files.get('image', False)
if not image:
return Response('input missing', status=400)
new_filename = str(uuid.uuid4()) + '.' + image.filename.split('.')[-1]
image.save(os.path.join(IMAGE_UPLOADS, new_filename))
print('saved: ' + new_filename)
return 'saved: ' + new_filename
On the client side:
<form action="/upload_img" method="post">
<input type="file" name="image">
<button type="submit">Submit</button>
</form>
If you use JavaScript to submit the request, make sure to use a POST
request with Content-Type: multipart/form-data